Package com.diozero.api
Class AnalogInputDevice
- All Implemented Interfaces:
DeviceEventConsumer<AnalogInputEvent>
,Closeable
,AutoCloseable
,Runnable
,Consumer<AnalogInputEvent>
- Direct Known Subclasses:
GP2Y0A21YK
,LDR
,Potentiometer
,TMP36
public class AnalogInputDevice extends GpioInputDevice<AnalogInputEvent> implements Runnable
The AnalogInputDevice base class encapsulates logic for interfacing with analog devices. This class provides access to unscaled (-1..1) and scaled (e.g. voltage, temperature, distance) readings. For scaled readings is important that the device factory is configured correctly - all raw analog readings are normalised (i.e. -1..1).
Note: The Raspberry Pi does not natively support analog input devices, see
McpAdc
for connecting to analog-to-digital
converters.
Example: Temperature readings using an MCP3008 and TMP36:
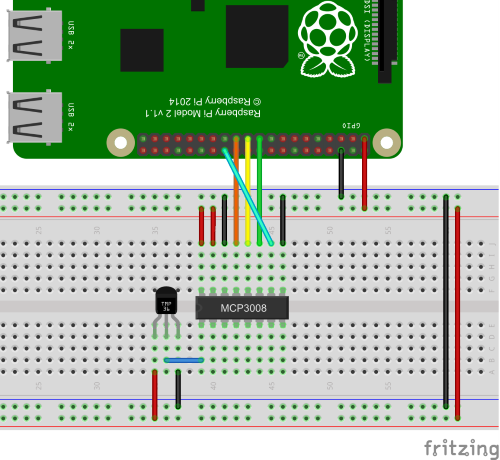
Code taken from TMP36Test:
try (McpAdc adc = new McpAdc(type, chipSelect);
TMP36 tmp36 = new TMP36(adc, pin, vRef, tempOffset)) {
for (int i=0; i<ITERATIONS; i++) {
double tmp = tmp36.getTemperature();
Logger.info("Temperature: {}", String.format("%.2f", Double.valueOf(tmp)));
SleepUtil.sleepSeconds(.5);
}
}
-
Field Summary
Fields inherited from class com.diozero.api.GpioDevice
gpio
-
Constructor Summary
Constructors Constructor Description AnalogInputDevice(int gpio)
AnalogInputDevice(int gpio, float range)
AnalogInputDevice(AnalogInputDeviceFactoryInterface deviceFactory, int gpio)
AnalogInputDevice(AnalogInputDeviceFactoryInterface deviceFactory, int gpio, float range)
-
Method Summary
Modifier and Type Method Description void
accept(AnalogInputEvent event)
void
addListener(DeviceEventConsumer<AnalogInputEvent> listener, float percentChange)
Register a listener for value changes, will check for changes every 50ms.void
addListener(DeviceEventConsumer<AnalogInputEvent> listener, float percentChange, int pollInterval)
Register a listener for value changes, will check for changes every 50ms.void
close()
float
convertToScaledValue(float unscaledValue)
Convert the specified unscaled value (-1..1) to a scaled one (-range..range).protected void
disableDeviceListener()
protected void
enableDeviceListener()
float
getRange()
Get the analog range for this input device as used bygetScaledValue()
andconvertToScaledValue(float)
float
getScaledValue()
Get the scaled value in the range 0..range (if unsigned) or -range..range (if signed)float
getUnscaledValue()
Get the unscaled normalised value in the range 0..1 (if unsigned) or -1..1 (if signed)void
run()
Methods inherited from class com.diozero.api.GpioInputDevice
addListener, removeAllListeners, removeListener
Methods inherited from class com.diozero.api.GpioDevice
getGpio
-
Constructor Details
-
AnalogInputDevice
- Parameters:
gpio
- GPIO to which the device is connected.- Throws:
RuntimeIOException
- If an I/O error occurred.
-
AnalogInputDevice
- Parameters:
gpio
- GPIO to which the device is connected.range
- To be used for taking scaled readings for this device.- Throws:
RuntimeIOException
- If an I/O error occurred.
-
AnalogInputDevice
public AnalogInputDevice(AnalogInputDeviceFactoryInterface deviceFactory, int gpio) throws RuntimeIOException- Parameters:
deviceFactory
- The device factory to use to provision this device.gpio
- GPIO to which the device is connected.- Throws:
RuntimeIOException
- If an I/O error occurred.
-
AnalogInputDevice
public AnalogInputDevice(AnalogInputDeviceFactoryInterface deviceFactory, int gpio, float range) throws RuntimeIOException- Parameters:
deviceFactory
- The device factory to use to provision this device.gpio
- GPIO to which the device is connected.range
- To be used for taking scaled readings for this device.- Throws:
RuntimeIOException
- If an I/O error occurred.
-
-
Method Details
-
close
- Specified by:
close
in interfaceAutoCloseable
- Specified by:
close
in interfaceCloseable
- Throws:
RuntimeIOException
-
enableDeviceListener
protected void enableDeviceListener()- Specified by:
enableDeviceListener
in classGpioInputDevice<AnalogInputEvent>
-
disableDeviceListener
protected void disableDeviceListener()- Specified by:
disableDeviceListener
in classGpioInputDevice<AnalogInputEvent>
-
run
public void run() -
accept
- Specified by:
accept
in interfaceConsumer<AnalogInputEvent>
- Overrides:
accept
in classGpioInputDevice<AnalogInputEvent>
-
getRange
public float getRange()Get the analog range for this input device as used bygetScaledValue()
andconvertToScaledValue(float)
- Returns:
- the analog range for this input device
-
getUnscaledValue
Get the unscaled normalised value in the range 0..1 (if unsigned) or -1..1 (if signed)- Returns:
- the unscaled value
- Throws:
RuntimeIOException
- if there was an I/O error
-
getScaledValue
Get the scaled value in the range 0..range (if unsigned) or -range..range (if signed)- Returns:
- the scaled value (-range..range)
- Throws:
RuntimeIOException
- if there was an I/O error
-
convertToScaledValue
public float convertToScaledValue(float unscaledValue)Convert the specified unscaled value (-1..1) to a scaled one (-range..range).- Parameters:
unscaledValue
- the unscaled value in the range -1..1- Returns:
- the scaled value in -range..range
- See Also:
getRange()
-
addListener
Register a listener for value changes, will check for changes every 50ms.- Parameters:
listener
- The listener callback.percentChange
- Degree of change required to trigger an event.
-
addListener
public void addListener(DeviceEventConsumer<AnalogInputEvent> listener, float percentChange, int pollInterval)Register a listener for value changes, will check for changes every 50ms.- Parameters:
listener
- The listener callback.percentChange
- Degree of change required to trigger an event.pollInterval
- Time in milliseconds at which reading should be taken.
-