Package com.diozero.devices
Class Button
- All Implemented Interfaces:
DeviceInterface
,DigitalInputDeviceInterface
,DeviceEventConsumer<DigitalInputEvent>
,AutoCloseable
,Consumer<DigitalInputEvent>
public class Button extends DigitalInputDevice
Provides push button related utility methods.
From the ButtonTest example:
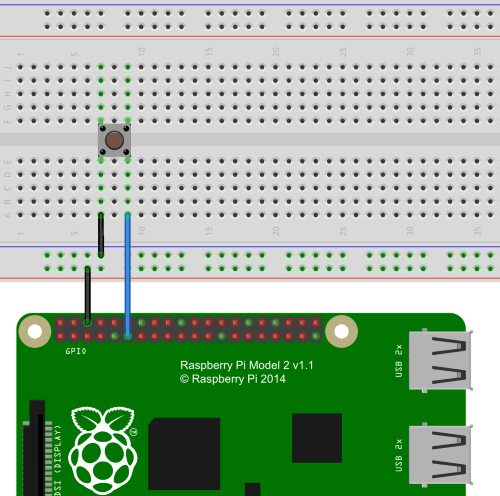
try (Button button = new Button(inputPin, GpioPullUpDown.PULL_UP)) {
button.addListener(event -> Logger.debug("Event: {}", event));
Logger.debug("Waiting for 10s - *** Press the button connected to input pin " + inputPin + " ***");
SleepUtil.sleepSeconds(10);
}
Controlling an LED with a button ButtonControlledLed:
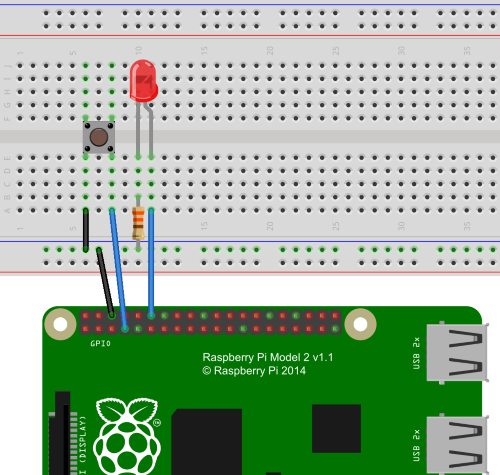
try (Button button = new Button(buttonPin, GpioPullUpDown.PULL_UP); LED led = new LED(ledPin)) {
button.whenPressed(nanoTime -> led::on);
button.whenReleased(nanoTime -> led::off);
Logger.info("Waiting for 10s - *** Press the button connected to pin {} ***", Integer.valueOf(buttonPin));
SleepUtil.sleepSeconds(10);
}
-
Nested Class Summary
Nested Classes Modifier and Type Class Description static class
Button.Builder
Button builder. -
Field Summary
Fields inherited from class com.diozero.api.AbstractDigitalInputDevice
activeHigh
Fields inherited from class com.diozero.api.GpioDevice
pinInfo
-
Constructor Summary
Constructors Constructor Description Button(int gpio)
Button(int gpio, GpioPullUpDown pud)
Button(int gpio, GpioPullUpDown pud, GpioEventTrigger trigger)
Button(GpioDeviceFactoryInterface deviceFactory, int gpio, GpioPullUpDown pud)
Button(GpioDeviceFactoryInterface deviceFactory, int gpio, GpioPullUpDown pud, GpioEventTrigger trigger)
Button(GpioDeviceFactoryInterface deviceFactory, PinInfo pinInfo, GpioPullUpDown pud, GpioEventTrigger trigger, boolean activeHigh)
-
Method Summary
Modifier and Type Method Description boolean
isPressed()
Get the current state.boolean
isReleased()
Get the current state.void
whenPressed(LongConsumer consumer)
Action to perform when the button is pressed.void
whenReleased(LongConsumer consumer)
Action to perform when the button is released.Methods inherited from class com.diozero.api.DigitalInputDevice
close, getPullUpDown, getTrigger, getValue, isActive, removeListener, setListener
Methods inherited from class com.diozero.api.AbstractDigitalInputDevice
accept, disableDeviceListener, enableDeviceListener, isActiveHigh, waitForActive, waitForActive, waitForInactive, waitForInactive, waitForValue, whenActivated, whenDeactivated
Methods inherited from class com.diozero.api.GpioInputDevice
addListener, hasListeners, removeAllListeners, removeListener
Methods inherited from class com.diozero.api.GpioDevice
getGpio
-
Constructor Details
-
Button
- Parameters:
gpio
- GPIO to which the device is connected.- Throws:
RuntimeIOException
- If an I/O error occurs.NoSuchDeviceException
-
Button
- Parameters:
gpio
- GPIO to which the button is connected.pud
- Pull up / down configuration (NONE, PULL_UP, PULL_DOWN).- Throws:
RuntimeIOException
- If an I/O error occurred.
-
Button
public Button(int gpio, GpioPullUpDown pud, GpioEventTrigger trigger) throws RuntimeIOException, NoSuchDeviceException- Parameters:
gpio
- GPIO to which the device is connectedpud
- Pull up/down configuration, values: NONE, PULL_UP, PULL_DOWNtrigger
- Event trigger configuration, values: NONE, RISING, FALLING, BOTH- Throws:
RuntimeIOException
- If an I/O error occursNoSuchDeviceException
-
Button
-
Button
public Button(GpioDeviceFactoryInterface deviceFactory, int gpio, GpioPullUpDown pud, GpioEventTrigger trigger) throws RuntimeIOException, NoSuchDeviceException- Parameters:
deviceFactory
- Device factory to use to provision this digital input devicegpio
- GPIO to which the device is connectedpud
- Pull up/down configuration, values: NONE, PULL_UP, PULL_DOWNtrigger
- Event trigger configuration, values: NONE, RISING, FALLING, BOTH- Throws:
RuntimeIOException
- If an I/O error occursNoSuchDeviceException
-
Button
public Button(GpioDeviceFactoryInterface deviceFactory, PinInfo pinInfo, GpioPullUpDown pud, GpioEventTrigger trigger, boolean activeHigh) throws RuntimeIOException, NoSuchDeviceException- Parameters:
deviceFactory
- Device factory to use to provision this digital input devicepinInfo
- Information about the GPIO pin to which the device is connectedpud
- Pull up/down configuration, values: NONE, PULL_UP, PULL_DOWNtrigger
- Event trigger configuration, values: NONE, RISING, FALLING, BOTHactiveHigh
- Set to true if digital 1 is to be treated as active- Throws:
RuntimeIOException
- If an I/O error occurs.NoSuchDeviceException
-
-
Method Details
-
isPressed
public boolean isPressed()Get the current state.- Returns:
- Return true if the button is currently pressed.
-
isReleased
public boolean isReleased()Get the current state.- Returns:
- Return true if the button is currently released.
-
whenPressed
Action to perform when the button is pressed.- Parameters:
consumer
- Callback function to invoke when pressed (long parameter is nanoseconds time).
-
whenReleased
Action to perform when the button is released.- Parameters:
consumer
- Callback function to invoke when pressed (long parameter is nanoseconds time).
-