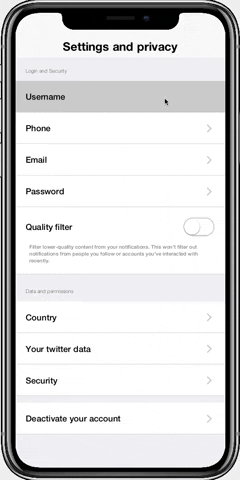
public class SettingsForm extends AbstractEntityView implements WidgetTypes
A settings form similar to the iOS and Android "Settings" applications.
package com.codename1.samples;
import static com.codename1.ui.CN.*;
import com.codename1.ui.Form;
import com.codename1.ui.Dialog;
import com.codename1.ui.plaf.UIManager;
import com.codename1.ui.util.Resources;
import com.codename1.io.Log;
import com.codename1.ui.Toolbar;
import com.codename1.rad.controllers.FormController;
import com.codename1.rad.models.BooleanProperty;
import com.codename1.rad.models.Entity;
import com.codename1.rad.models.EntityType;
import static com.codename1.rad.models.EntityType.description;
import static com.codename1.rad.models.EntityType.tags;
import com.codename1.rad.models.PropertySelector;
import com.codename1.rad.nodes.ActionNode;
import com.codename1.rad.nodes.ViewNode;
import com.codename1.rad.schemas.Person;
import com.codename1.rad.schemas.PostalAddress;
import com.codename1.rad.schemas.Thing;
import com.codename1.rad.ui.SettingsForm;
import static com.codename1.rad.ui.UI.*;
import com.codename1.ui.Button;
import com.codename1.ui.layouts.BoxLayout;
import com.codename1.ui.list.DefaultListModel;
/**
* This sample demonstrates the use of the SettingsForm class edit properties of an Entity, using
* the CodeRAD cn1lib.
* */
public class SettingsFormSample {
/**
* Define the actions to include in the settings form
* */
public static final ActionNode
/**
* A username field.
*/
username = action(
label("Username"),
/**
* By adding a property to the action, the SettingsForm will
* display the property value on the action.
*/
property(entity->{
return new PropertySelector(entity, Thing.identifier);
}),
/**
* We make the username action editable by adding a text field
*/
textField(
label("Enter Username"),
description("Please enter a new username below"),
tags(Thing.identifier)
)
),
/**
* A sample "switch" widget
*/
qualityFilter = action(
label("Quality filter"),
/**
* This will be rendered as a toggle switch in the settings form
* because we include the toggleSwitch() node here.
*/
toggleSwitch(
/**
* The property that the toggle switch should be bound to.
*/
property(UserProfile.qualityFilter),
description("Filter lower-quality content from your notifications. This won't filter out notifications from people you follow or accounts you've interacted with recently.")
)
),
/**
* A phone action. In this sample we bind this to the "telephone" property,
* but we don't make it editable directly. The intention is that we'll
* add a custom action listener for this action and handle it in our own way.
*/
phone = action(
label("Phone"),
/**
* Display the telephone property on the menu item.
*/
tags(Person.telephone)
),
email = action(
label("Email"),
tags(Person.email)
),
password = action(
label("Password")
),
/**
* An action to display and change the "country" of the entity.
*/
country = action(
label("Country"),
/**
* Use a radio list with BoxLayout.Y layout to change the country
* selection.
*/
radioListY(
label("Select Country"),
description("Please select a country from the list below"),
options(new DefaultListModel("Canada", "United States", "Mexico", "Spain", "England", "France")),
tags(PostalAddress.addressCountry)
)
),
yourTwitterData = action(
label("Your twitter data")
),
security = action(
label("Security")
),
deactivate = action(
label("Deactivate your account")
);
private Form current;
private Resources theme;
public void init(Object context) {
// use two network threads instead of one
updateNetworkThreadCount(2);
theme = UIManager.initFirstTheme("/theme");
// Enable Toolbar on all Forms by default
Toolbar.setGlobalToolbar(true);
// Pro only feature
Log.bindCrashProtection(true);
addNetworkErrorListener(err -> {
// prevent the event from propagating
err.consume();
if(err.getError() != null) {
Log.e(err.getError());
}
Log.sendLogAsync();
Dialog.show("Connection Error", "There was a networking error in the connection to " + err.getConnectionRequest().getUrl(), "OK", null);
});
}
public void start() {
if(current != null){
current.show();
return;
}
Form f = new Form(BoxLayout.y());
Button b = new Button("Open Settings");
b.addActionListener(e->{
new SettingsFormController().getView().show();
});
f.add(b);
f.show();
}
public void stop() {
current = getCurrentForm();
if(current instanceof Dialog) {
((Dialog)current).dispose();
current = getCurrentForm();
}
}
public void destroy() {
}
public class SettingsFormController extends FormController {
public SettingsFormController() {
super(null);
// Create the view model
UserProfile profile = new UserProfile();
// Create the view
SettingsForm view = new SettingsForm(profile, getViewNode());
// Set the title
setTitle("Settings and privacy");
// Set the view. This will be wrapped in a form.
setView(view);
}
/**
* This method creates the View node that is used for building views with this
* controller. The view node we build here is designed to be used by the
* SettingsForm view to generate a settings form. The SettingsForm expects
* a list of SectionNodes, each with its only list of FieldNodes.
* @return
*/
@Override
protected ViewNode createViewNode() {
return new ViewNode(
section(
label("Login and Security"),
username, phone, email, password, qualityFilter
),
section(
label("Data and permissions"),
country, yourTwitterData, security
),
section(
deactivate
)
);
}
}
/**
* A dummy entity we use for this sample. Represents a user profile.
*/
public static class UserProfile extends Entity {
public static BooleanProperty qualityFilter;
private static final EntityType TYPE = new EntityType() {{
/**
* The "name" field.
*/
string(tags(Thing.name));
/**
* The "id" field
*/
string(tags(Thing.identifier));
/**
* The thumbnailUrl field
*/
string(tags(Thing.thumbnailUrl));
/**
* The "country" field.
*/
string(tags(PostalAddress.addressCountry));
/**
* The qualityFilter field. We don't use tags on this one because
* this property doesn't have any corresponding generic tag that would
* apply.
*/
qualityFilter = Boolean();
}};
{
/**
* For entities we always need to set the entity type in the initializer.
*/
setEntityType(TYPE);
}
}
}
ViewNode
FormatThe view node used to build the settings form should contain zero or more SectionNode
children, which
will be rendered as sections of the settings form using SettingsForm.SettingsFormSection
. Each section should include
one or more ActionNode
child nodes, which will be rendered as SettingsForm.SettingsFormActionView
.
See the docs for SettingsForm.SettingsFormActionView
for details on the expected format of each ActionNode
.
The complete example above uses the following view node:
new ViewNode(
section(
label("Login and Security"),
username, phone, email, password, qualityFilter
),
section(
label("Data and permissions"),
country, yourTwitterData, security
),
section(
deactivate
)
);
In this example, the settings form is split into 30 sections, "Login and Security", "Data and Permissions", and a last section that has no heading.
SettingsForm
- UIID for the SettingForm component itself.
See SettingsForm.SettingsFormSection
and SettingsForm.SettingsFormActionView
documentation for styles used in the subcomponents.
If you add the UIIDPrefix
attribute to the ViewNode, that prefix will be added to all UIIDs in this component and subcomponents.
Modifier and Type | Class and Description |
---|---|
static class |
SettingsForm.SettingsFormActionView
A view for rendering an
ActionNode as a menu item in SettingsForm . |
static class |
SettingsForm.SettingsFormActionViewFactory
ViewFactory for rendering actions on
SettingsForm . |
static class |
SettingsForm.SettingsFormSection
Container used to render a section of
SettingsForm . |
BASELINE, BOTTOM, BRB_CENTER_OFFSET, BRB_CONSTANT_ASCENT, BRB_CONSTANT_DESCENT, BRB_OTHER, CENTER, CROSSHAIR_CURSOR, DEFAULT_CURSOR, DRAG_REGION_IMMEDIATELY_DRAG_X, DRAG_REGION_IMMEDIATELY_DRAG_XY, DRAG_REGION_IMMEDIATELY_DRAG_Y, DRAG_REGION_LIKELY_DRAG_X, DRAG_REGION_LIKELY_DRAG_XY, DRAG_REGION_LIKELY_DRAG_Y, DRAG_REGION_NOT_DRAGGABLE, DRAG_REGION_POSSIBLE_DRAG_X, DRAG_REGION_POSSIBLE_DRAG_XY, DRAG_REGION_POSSIBLE_DRAG_Y, E_RESIZE_CURSOR, HAND_CURSOR, LEFT, MOVE_CURSOR, N_RESIZE_CURSOR, NE_RESIZE_CURSOR, NW_RESIZE_CURSOR, RIGHT, S_RESIZE_CURSOR, SE_RESIZE_CURSOR, SW_RESIZE_CURSOR, TEXT_CURSOR, TOP, W_RESIZE_CURSOR, WAIT_CURSOR
CHECKBOX, CHECKBOX_LIST, COMBOBOX, HTML_COMPONENT, RADIO, RADIO_LIST, SWITCH, SWITCH_LIST, TABLE, TEXT, TEXTAREA
Constructor and Description |
---|
SettingsForm(Entity entity,
ViewNode node)
Creates a new SettingsForm to edit properties of the given entity.
|
Modifier and Type | Method and Description |
---|---|
void |
commit() |
Node |
getViewNode() |
void |
update() |
bind, bindImpl, deinitialize, findProperty, getEntity, initComponent, isBindOnPropertyChangeEvents, setBindOnPropertyChangeEvents, setEntity, unbind, unbindImpl
add, add, add, add, add, add, addAll, addComponent, addComponent, addComponent, addComponent, animateHierarchy, animateHierarchyAndWait, animateHierarchyFade, animateHierarchyFadeAndWait, animateLayout, animateLayoutAndWait, animateLayoutFade, animateLayoutFadeAndWait, animateUnlayout, animateUnlayoutAndWait, applyRTL, calcPreferredSize, cancelRepaints, clearClientProperties, constrainHeightWhenScrollable, constrainWidthWhenScrollable, contains, createAnimateHierarchy, createAnimateHierarchyFade, createAnimateLayout, createAnimateLayoutFade, createAnimateLayoutFadeAndWait, createAnimateMotion, createAnimateUnlayout, createReplaceTransition, dragInitiated, drop, encloseIn, encloseIn, findDropTargetAt, findFirstFocusable, fireClicked, flushReplace, forceRevalidate, getBottomGap, getChildrenAsList, getClosestComponentTo, getComponentAt, getComponentAt, getComponentCount, getComponentIndex, getGridPosX, getGridPosY, getLayout, getLayoutHeight, getLayoutWidth, getLeadComponent, getLeadParent, getResponderAt, getSafeAreaRoot, getScrollIncrement, getSideGap, getUIManager, initLaf, invalidate, isEnabled, isSafeArea, isSafeAreaRoot, isScrollableX, isScrollableY, isSelectableInteraction, iterator, iterator, keyPressed, keyReleased, layoutContainer, morph, morphAndWait, paint, paintComponentBackground, paintGlass, paramString, pointerPressed, refreshTheme, removeAll, removeComponent, replace, replace, replaceAndWait, replaceAndWait, replaceAndWait, revalidate, revalidateLater, revalidateWithAnimationSafety, scrollComponentToVisible, setCellRenderer, setEnabled, setLayout, setLeadComponent, setSafeArea, setSafeAreaRoot, setScrollable, setScrollableX, setScrollableY, setScrollIncrement, setShouldCalcPreferredSize, setShouldLayout, setUIManager, updateTabIndices
addDragFinishedListener, addDragOverListener, addDropListener, addFocusListener, addLongPressListener, addPointerDraggedListener, addPointerPressedListener, addPointerReleasedListener, addPullToRefresh, addScrollListener, addStateChangeListener, animate, bindProperty, blocksSideSwipe, calcScrollSize, contains, containsOrOwns, createStyleAnimation, deinitializeCustomStyle, dragEnter, dragExit, dragFinished, draggingOver, drawDraggedImage, focusGained, focusLost, getAbsoluteX, getAbsoluteY, getAllStyles, getAnimationManager, getBaseline, getBaselineResizeBehavior, getBindablePropertyNames, getBindablePropertyTypes, getBorder, getBoundPropertyValue, getBounds, getBounds, getClientProperty, getCloudBoundProperty, getCloudDestinationProperty, getComponentForm, getComponentState, getCursor, getDirtyRegion, getDisabledStyle, getDraggedx, getDraggedy, getDragImage, getDragRegionStatus, getDragSpeed, getEditingDelegate, getHeight, getInlineAllStyles, getInlineDisabledStyles, getInlinePressedStyles, getInlineSelectedStyles, getInlineStylesTheme, getInlineUnselectedStyles, getInnerHeight, getInnerPreferredH, getInnerPreferredW, getInnerWidth, getInnerX, getInnerY, getLabelForComponent, getName, getNativeOverlay, getNextFocusDown, getNextFocusLeft, getNextFocusRight, getNextFocusUp, getOuterHeight, getOuterPreferredH, getOuterPreferredW, getOuterWidth, getOuterX, getOuterY, getOwner, getParent, getPreferredH, getPreferredSize, getPreferredSizeStr, getPreferredTabIndex, getPreferredW, getPressedStyle, getPropertyNames, getPropertyTypeNames, getPropertyTypes, getPropertyValue, getSameHeight, getSameWidth, getScrollable, getScrollAnimationSpeed, getScrollDimension, getScrollOpacity, getScrollOpacityChangeSpeed, getScrollX, getScrollY, getSelectCommandText, getSelectedRect, getSelectedStyle, getStyle, getTabIndex, getTensileLength, getTextSelectionSupport, getTooltip, getUIID, getUnselectedStyle, getVisibleBounds, getVisibleBounds, getWidth, getX, getY, growShrink, handlesInput, hasFixedPreferredSize, hasFocus, hideNativeOverlay, initCustomStyle, installDefaultPainter, isAlwaysTensile, isBlockLead, isCellRenderer, isChildOf, isDragActivated, isDragAndDropOperation, isDraggable, isDragRegion, isDropTarget, isEditable, isEditing, isFlatten, isFocusable, isGrabsPointerEvents, isHidden, isHidden, isHideInLandscape, isHideInPortrait, isIgnorePointerEvents, isInClippingRegion, isInitialized, isOpaque, isOwnedBy, isRippleEffect, isRTL, isScrollable, isScrollVisible, isSetCursorSupported, isSmoothScrolling, isSnapToGrid, isStickyDrag, isTactileTouch, isTactileTouch, isTensileDragEnabled, isTraversable, isVisible, keyRepeated, laidOut, longKeyPress, longPointerPress, onScrollX, onScrollY, paintBackground, paintBackgrounds, paintBorder, paintBorderBackground, paintComponent, paintComponent, paintIntersectingComponentsAbove, paintLock, paintLockRelease, paintRippleOverlay, paintScrollbars, paintScrollbarX, paintScrollbarY, parsePreferredSize, pinch, pinchReleased, pointerDragged, pointerDragged, pointerHover, pointerHoverPressed, pointerHoverReleased, pointerPressed, pointerReleased, pointerReleased, putClientProperty, refreshTheme, refreshTheme, remove, removeDragFinishedListener, removeDragOverListener, removeDropListener, removeFocusListener, removeLongPressListener, removePointerDraggedListener, removePointerPressedListener, removePointerReleasedListener, removeScrollListener, removeStateChangeListener, repaint, repaint, requestFocus, resetFocusable, respondsToPointerEvents, scrollRectToVisible, scrollRectToVisible, setAlwaysTensile, setBlockLead, setBoundPropertyValue, setCloudBoundProperty, setCloudDestinationProperty, setComponentState, setCursor, setDirtyRegion, setDisabledStyle, setDraggable, setDropTarget, setEditingDelegate, setFlatten, setFocus, setFocusable, setGrabsPointerEvents, setHandlesInput, setHeight, setHidden, setHidden, setHideInLandscape, setHideInPortrait, setIgnorePointerEvents, setInitialized, setInlineAllStyles, setInlineDisabledStyles, setInlinePressedStyles, setInlineSelectedStyles, setInlineStylesTheme, setInlineUnselectedStyles, setIsScrollVisible, setLabelForComponent, setName, setNextFocusDown, setNextFocusLeft, setNextFocusRight, setNextFocusUp, setOpaque, setOwner, setPreferredH, setPreferredSize, setPreferredSizeStr, setPreferredTabIndex, setPreferredW, setPressedStyle, setPropertyValue, setRippleEffect, setRTL, setSameHeight, setSameSize, setSameWidth, setScrollAnimationSpeed, setScrollOpacityChangeSpeed, setScrollSize, setScrollVisible, setScrollX, setScrollY, setSelectCommandText, setSelectedStyle, setSize, setSmoothScrolling, setSnapToGrid, setTabIndex, setTactileTouch, setTensileDragEnabled, setTensileLength, setTooltip, setTraversable, setUIID, setUIID, setUnselectedStyle, setVisible, setWidth, setX, setY, shouldBlockSideSwipe, shouldRenderComponentSelection, showNativeOverlay, startEditingAsync, stopEditing, stripMarginAndPadding, styleChanged, toImage, toString, unbindProperty, updateNativeOverlay, visibleBoundsContains
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
forEach, spliterator
public void update()
update
in interface EntityView
public void commit()
commit
in interface EntityView
public Node getViewNode()
getViewNode
in interface EntityView
Copyright © 2021. All Rights Reserved.