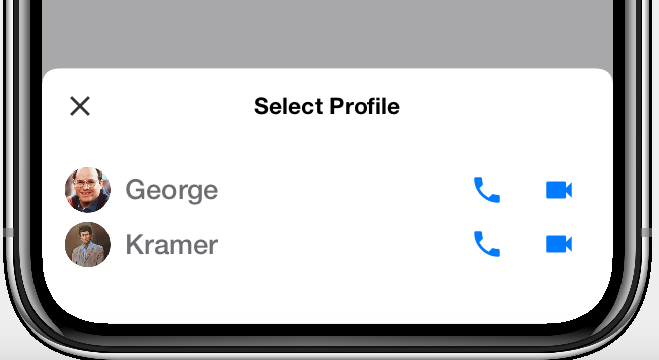
public class ProfileListView extends EntityListView
A list view that can show a list of profiles.
Each row shows the profile avatar/icon,
name, and optionally a set of actions that will be rendered as buttons for the user to perform. Row click
events can be handled by registering an action in the ACCOUNT_LIST_ROW_SELECTED
category. Row actions
can be assigned to the ACCOUNT_LIST_ROW_ACTIONS
category.
This view will accept any EntityList
where the row items are "profiles". For best results, each profile
should contain a name and icon, although it will fall back to suitable output if one or both of those fields are missing.
The icon is rendered using the ProfileAvatarView
view, so see its docs for details on what information it needs. A simple
solution, that will always work, is to make sure that the profile entities contain properties tagged with Thing.name
and Thing.thumbnailUrl
.
Since rows are rendered using ProfileListView.ProfileListRowView
, you can also see its docs for information about row view model requirements.
The following actions are supported by this view:
Modifier and Type | Class and Description |
---|---|
static class |
ProfileListView.ProfileListRowCellRenderer
A list cell renderer for rendering rows of a list with
ProfileListView.ProfileListRowView . |
static class |
ProfileListView.ProfileListRowView
Row view for rendering a single row of the
ProfileListView . |
EntityListView.RowLayout
Modifier and Type | Field and Description |
---|---|
static ActionNode.Category |
ACCOUNT_LIST_ROW_ACTIONS
Actions that can be performed on a single row of the list.
|
static ActionNode.Category |
ACCOUNT_LIST_ROW_SELECTED
Category used to register an action to receive "click" events for a row.
|
ANIMATE_INSERTIONS, ANIMATE_REMOVALS, COLUMNS, LANDSCAPE_COLUMNS, LAYOUT, SCROLLABLE_X, SCROLLABLE_Y
BASELINE, BOTTOM, BRB_CENTER_OFFSET, BRB_CONSTANT_ASCENT, BRB_CONSTANT_DESCENT, BRB_OTHER, CENTER, CROSSHAIR_CURSOR, DEFAULT_CURSOR, DRAG_REGION_IMMEDIATELY_DRAG_X, DRAG_REGION_IMMEDIATELY_DRAG_XY, DRAG_REGION_IMMEDIATELY_DRAG_Y, DRAG_REGION_LIKELY_DRAG_X, DRAG_REGION_LIKELY_DRAG_XY, DRAG_REGION_LIKELY_DRAG_Y, DRAG_REGION_NOT_DRAGGABLE, DRAG_REGION_POSSIBLE_DRAG_X, DRAG_REGION_POSSIBLE_DRAG_XY, DRAG_REGION_POSSIBLE_DRAG_Y, E_RESIZE_CURSOR, HAND_CURSOR, LEFT, MOVE_CURSOR, N_RESIZE_CURSOR, NE_RESIZE_CURSOR, NW_RESIZE_CURSOR, RIGHT, S_RESIZE_CURSOR, SE_RESIZE_CURSOR, SW_RESIZE_CURSOR, TEXT_CURSOR, TOP, W_RESIZE_CURSOR, WAIT_CURSOR
Constructor and Description |
---|
ProfileListView(EntityList list) |
ProfileListView(EntityList list,
ListNode node,
float avatarSizeMM) |
bindImpl, commit, getListCellRenderer, getListLayout, getRowViewForEntity, getScrollWrapper, getVerticalScroller, getViewNode, isAnimateInsertions, isAnimateRemovals, setAnimateInsertions, setAnimateRemovals, setListCellRenderer, setListLayout, setScrollableY, unbindImpl, update
bind, deinitialize, findProperty, getEntity, initComponent, isBindOnPropertyChangeEvents, setBindOnPropertyChangeEvents, setEntity, unbind
add, add, add, add, add, add, addAll, addComponent, addComponent, addComponent, addComponent, animateHierarchy, animateHierarchyAndWait, animateHierarchyFade, animateHierarchyFadeAndWait, animateLayout, animateLayoutAndWait, animateLayoutFade, animateLayoutFadeAndWait, animateUnlayout, animateUnlayoutAndWait, applyRTL, calcPreferredSize, cancelRepaints, clearClientProperties, constrainHeightWhenScrollable, constrainWidthWhenScrollable, contains, createAnimateHierarchy, createAnimateHierarchyFade, createAnimateLayout, createAnimateLayoutFade, createAnimateLayoutFadeAndWait, createAnimateMotion, createAnimateUnlayout, createReplaceTransition, dragInitiated, drop, encloseIn, encloseIn, findDropTargetAt, findFirstFocusable, fireClicked, flushReplace, forceRevalidate, getBottomGap, getChildrenAsList, getClosestComponentTo, getComponentAt, getComponentAt, getComponentCount, getComponentIndex, getGridPosX, getGridPosY, getLayout, getLayoutHeight, getLayoutWidth, getLeadComponent, getLeadParent, getResponderAt, getSafeAreaRoot, getScrollIncrement, getSideGap, getUIManager, initLaf, invalidate, isEnabled, isSafeArea, isSafeAreaRoot, isScrollableX, isScrollableY, isSelectableInteraction, iterator, iterator, keyPressed, keyReleased, layoutContainer, morph, morphAndWait, paint, paintComponentBackground, paintGlass, paramString, pointerPressed, refreshTheme, removeAll, removeComponent, replace, replace, replaceAndWait, replaceAndWait, replaceAndWait, revalidate, revalidateLater, revalidateWithAnimationSafety, scrollComponentToVisible, setCellRenderer, setEnabled, setLayout, setLeadComponent, setSafeArea, setSafeAreaRoot, setScrollable, setScrollableX, setScrollIncrement, setShouldCalcPreferredSize, setShouldLayout, setUIManager, updateTabIndices
addDragFinishedListener, addDragOverListener, addDropListener, addFocusListener, addLongPressListener, addPointerDraggedListener, addPointerPressedListener, addPointerReleasedListener, addPullToRefresh, addScrollListener, addStateChangeListener, animate, bindProperty, blocksSideSwipe, calcScrollSize, contains, containsOrOwns, createStyleAnimation, deinitializeCustomStyle, dragEnter, dragExit, dragFinished, draggingOver, drawDraggedImage, focusGained, focusLost, getAbsoluteX, getAbsoluteY, getAllStyles, getAnimationManager, getBaseline, getBaselineResizeBehavior, getBindablePropertyNames, getBindablePropertyTypes, getBorder, getBoundPropertyValue, getBounds, getBounds, getClientProperty, getCloudBoundProperty, getCloudDestinationProperty, getComponentForm, getComponentState, getCursor, getDirtyRegion, getDisabledStyle, getDraggedx, getDraggedy, getDragImage, getDragRegionStatus, getDragSpeed, getEditingDelegate, getHeight, getInlineAllStyles, getInlineDisabledStyles, getInlinePressedStyles, getInlineSelectedStyles, getInlineStylesTheme, getInlineUnselectedStyles, getInnerHeight, getInnerPreferredH, getInnerPreferredW, getInnerWidth, getInnerX, getInnerY, getLabelForComponent, getName, getNativeOverlay, getNextFocusDown, getNextFocusLeft, getNextFocusRight, getNextFocusUp, getOuterHeight, getOuterPreferredH, getOuterPreferredW, getOuterWidth, getOuterX, getOuterY, getOwner, getParent, getPreferredH, getPreferredSize, getPreferredSizeStr, getPreferredTabIndex, getPreferredW, getPressedStyle, getPropertyNames, getPropertyTypeNames, getPropertyTypes, getPropertyValue, getSameHeight, getSameWidth, getScrollable, getScrollAnimationSpeed, getScrollDimension, getScrollOpacity, getScrollOpacityChangeSpeed, getScrollX, getScrollY, getSelectCommandText, getSelectedRect, getSelectedStyle, getStyle, getTabIndex, getTensileLength, getTextSelectionSupport, getTooltip, getUIID, getUnselectedStyle, getVisibleBounds, getVisibleBounds, getWidth, getX, getY, growShrink, handlesInput, hasFixedPreferredSize, hasFocus, hideNativeOverlay, initCustomStyle, installDefaultPainter, isAlwaysTensile, isBlockLead, isCellRenderer, isChildOf, isDragActivated, isDragAndDropOperation, isDraggable, isDragRegion, isDropTarget, isEditable, isEditing, isFlatten, isFocusable, isGrabsPointerEvents, isHidden, isHidden, isHideInLandscape, isHideInPortrait, isIgnorePointerEvents, isInClippingRegion, isInitialized, isOpaque, isOwnedBy, isRippleEffect, isRTL, isScrollable, isScrollVisible, isSetCursorSupported, isSmoothScrolling, isSnapToGrid, isStickyDrag, isTactileTouch, isTactileTouch, isTensileDragEnabled, isTraversable, isVisible, keyRepeated, laidOut, longKeyPress, longPointerPress, onScrollX, onScrollY, paintBackground, paintBackgrounds, paintBorder, paintBorderBackground, paintComponent, paintComponent, paintIntersectingComponentsAbove, paintLock, paintLockRelease, paintRippleOverlay, paintScrollbars, paintScrollbarX, paintScrollbarY, parsePreferredSize, pinch, pinchReleased, pointerDragged, pointerDragged, pointerHover, pointerHoverPressed, pointerHoverReleased, pointerPressed, pointerReleased, pointerReleased, putClientProperty, refreshTheme, refreshTheme, remove, removeDragFinishedListener, removeDragOverListener, removeDropListener, removeFocusListener, removeLongPressListener, removePointerDraggedListener, removePointerPressedListener, removePointerReleasedListener, removeScrollListener, removeStateChangeListener, repaint, repaint, requestFocus, resetFocusable, respondsToPointerEvents, scrollRectToVisible, scrollRectToVisible, setAlwaysTensile, setBlockLead, setBoundPropertyValue, setCloudBoundProperty, setCloudDestinationProperty, setComponentState, setCursor, setDirtyRegion, setDisabledStyle, setDraggable, setDropTarget, setEditingDelegate, setFlatten, setFocus, setFocusable, setGrabsPointerEvents, setHandlesInput, setHeight, setHidden, setHidden, setHideInLandscape, setHideInPortrait, setIgnorePointerEvents, setInitialized, setInlineAllStyles, setInlineDisabledStyles, setInlinePressedStyles, setInlineSelectedStyles, setInlineStylesTheme, setInlineUnselectedStyles, setIsScrollVisible, setLabelForComponent, setName, setNextFocusDown, setNextFocusLeft, setNextFocusRight, setNextFocusUp, setOpaque, setOwner, setPreferredH, setPreferredSize, setPreferredSizeStr, setPreferredTabIndex, setPreferredW, setPressedStyle, setPropertyValue, setRippleEffect, setRTL, setSameHeight, setSameSize, setSameWidth, setScrollAnimationSpeed, setScrollOpacityChangeSpeed, setScrollSize, setScrollVisible, setScrollX, setScrollY, setSelectCommandText, setSelectedStyle, setSize, setSmoothScrolling, setSnapToGrid, setTabIndex, setTactileTouch, setTensileDragEnabled, setTensileLength, setTooltip, setTraversable, setUIID, setUIID, setUnselectedStyle, setVisible, setWidth, setX, setY, shouldBlockSideSwipe, shouldRenderComponentSelection, showNativeOverlay, startEditingAsync, stopEditing, stripMarginAndPadding, styleChanged, toImage, toString, unbindProperty, updateNativeOverlay, visibleBoundsContains
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
forEach, spliterator
public static final ActionNode.Category ACCOUNT_LIST_ROW_ACTIONS
Actions that can be performed on a single row of the list. These will be rendered in the right side of the row, as buttons.
public static final ActionNode.Category ACCOUNT_LIST_ROW_SELECTED
Category used to register an action to receive "click" events for a row.
public ProfileListView(EntityList list)
public ProfileListView(EntityList list, ListNode node, float avatarSizeMM)
Copyright © 2021. All Rights Reserved.