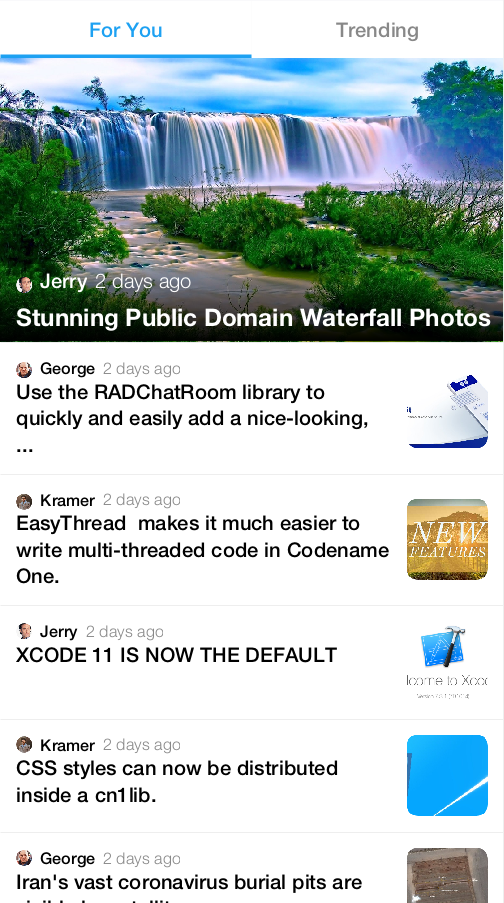
public class TabsEntityView extends AbstractEntityView implements CollapsibleHeaderContainer.ScrollableContainer
An Tabs component that is backed by a view model. You can specify the tabs in this view using the UI descriptor.
The view model does not need to conform necessarily to any particular schema, as the UI descriptor is fully responsible for the contents of the tabs. The UI descriptor may refer to elements of the view model for defining its contents though.
The UI descriptor is expected to be a ViewNode
, where its child nodes each correspond to a
tab in this tab component. Each child node may be either a ListNode
or a ViewNode
.
The tab label should be specified by the Property.Label
attribute. The tab contents will be defined
by the EntityViewFactory
for a view node, and the EntityListCellRenderer
for a list
node. They should also each specify either a property
or tags
attribute that points to the view
model, to retrieve the "sub" view model that should be used for the particular tab.
ViewNode vn = new ViewNode(
list(
property(SearchTabsViewModel.forYou),
label("For You"),
cellRenderer(new TWTNewsList.TWTNewsListCellRenderer())
),
list(
property(SearchTabsViewModel.trending),
label("Trending"),
cellRenderer(new TWTNewsList.TWTNewsListCellRenderer())
),
actions(TWTSearchButton.SEARCH_ACTION, search)
);
public static class SearchTabsViewModel extends Entity {
public static Property forYou, trending, news, sports, fun;
public static final EntityType TYPE = new EntityType() {{
forYou = list(EntityList.class);
trending = list(EntityList.class);
news = list(EntityList.class);
sports = list(EntityList.class);
fun = list(EntityList.class);
}};
{
setEntityType(TYPE);
}
}
both tabs are list tabs.
package com.codename1.demos.twitterui;
import ca.weblite.shared.components.CollapsibleHeaderContainer;
import com.codename1.demos.twitterui.models.NewsItem;
import com.codename1.demos.twitterui.models.UserProfile;
import com.codename1.rad.controllers.Controller;
import com.codename1.rad.models.Entity;
import com.codename1.rad.models.EntityList;
import com.codename1.rad.models.EntityType;
import com.codename1.rad.models.Property;
import com.codename1.rad.nodes.ActionNode;
import com.codename1.rad.nodes.ViewNode;
import com.codename1.rad.schemas.Thing;
import com.codename1.twitterui.models.TWTAuthor;
import com.codename1.twitterui.schemas.TWTNewsItem;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import static com.codename1.rad.ui.UI.*;
import com.codename1.rad.ui.entityviews.TabsEntityView;
import com.codename1.twitterui.views.TWTNewsList;
import com.codename1.twitterui.views.TWTSearchButton;
import com.codename1.twitterui.views.TWTTabsView;
import com.codename1.twitterui.views.TWTTitleComponent;
import com.codename1.ui.Form;
public class SearchTabsController extends BaseFormController {
public static final ActionNode search = action(
);
private Map<String,Entity> authors = new HashMap<>();
public SearchTabsController(Controller parent) {
super(parent);
SearchTabsViewModel model = createViewModel();
addLookup(model);
TabsEntityView view = new TWTTabsView(model, getViewNode());
CollapsibleHeaderContainer wrapper = new CollapsibleHeaderContainer(
new TWTTitleComponent(lookup(UserProfile.class), getViewNode(), new TWTSearchButton(lookup(UserProfile.class), getViewNode())),
view,
view
);
setView(wrapper);
addActionListener(search, evt->{
evt.consume();
Form form = new SearchFormController(this).getView();
form.show();
});
}
@Override
protected ViewNode createViewNode() {
ViewNode vn = new ViewNode(
list(
property(SearchTabsViewModel.forYou),
label("For You"),
cellRenderer(new TWTNewsList.TWTNewsListCellRenderer())
),
list(
property(SearchTabsViewModel.trending),
label("Trending"),
cellRenderer(new TWTNewsList.TWTNewsListCellRenderer())
),
actions(TWTSearchButton.SEARCH_ACTION, search)
);
return vn;
}
public static class SearchTabsViewModel extends Entity {
public static Property forYou, trending, news, sports, fun;
public static final EntityType TYPE = new EntityType() {{
forYou = list(EntityList.class);
trending = list(EntityList.class);
news = list(EntityList.class);
sports = list(EntityList.class);
fun = list(EntityList.class);
}};
{
setEntityType(TYPE);
}
}
private NewsItem createItem(Entity creator, Date date, String headline, String thumbnailUrl) {
NewsItem item = new NewsItem();
item.setEntity(TWTNewsItem.creator, creator);
item.setDate(TWTNewsItem.date, date);
item.setText(TWTNewsItem.headline, headline);
item.setText(TWTNewsItem.thumbnailUrl, thumbnailUrl);
item.setText(TWTNewsItem.image, thumbnailUrl);
return item;
}
private EntityList createSection() {
EntityList out = new EntityList();
String georgeThumb = "https://weblite.ca/cn1tests/radchat/george.jpg";
String kramerThumb = "https://weblite.ca/cn1tests/radchat/kramer.jpg";
String jerryThumb = "https://weblite.ca/cn1tests/radchat/jerry.jpg";
Entity george = getOrCreateAuthor("George", "@kostanza", georgeThumb);
Entity kramer = getOrCreateAuthor("Kramer", "@kramer", kramerThumb);
Entity jerry = getOrCreateAuthor("Jerry", "@jerry", jerryThumb);
long SECOND = 1000l;
long MINUTE = SECOND * 60;
long HOUR = MINUTE * 60;
long DAY = HOUR * 24;
long t = System.currentTimeMillis() - 2 * DAY;
out.add(createItem(jerry, new Date(t), "Stunning Public Domain Waterfall Photos", "https://weblite.ca/cn1tests/radchat/waterfalls.jpg"));
out.add(createItem(george, new Date(t), "Use the RADChatRoom library to quickly and easily add a nice-looking, ...", "https://www.codenameone.com/img/blog/chat-ui-kit-feature.jpg"));
out.add(createItem(kramer, new Date(t), "EasyThread makes it much easier to write multi-threaded code in Codename One.", "https://www.codenameone.com/img/blog/new-features.jpg"));
out.add(createItem(jerry, new Date(t), "XCODE 11 IS NOW THE DEFAULT", "https://www.codenameone.com/img/blog/xcode-7.png"));
out.add(createItem(kramer, new Date(t), "CSS styles can now be distributed inside a cn1lib.", "https://www.codenameone.com/img/blog/css-header.jpg"));
out.add(createItem(george, new Date(t), "Iran's vast coronavirus burial pits are visible by satellite", "https://pbs.twimg.com/media/ES6gV-xXkAQwZ0Y?format=jpg&name=small"));
out.add(createItem(george, new Date(t), "We've added the ability to position your Sheets in different locations on the screen.", "https://www.codenameone.com/img/blog/new-features.jpg"));
return out;
}
private EntityList createTrendingSection() {
EntityList out = new EntityList();
String georgeThumb = "https://weblite.ca/cn1tests/radchat/george.jpg";
String kramerThumb = "https://weblite.ca/cn1tests/radchat/kramer.jpg";
Entity george = getOrCreateAuthor("George", "@kostanza", georgeThumb);
Entity kramer = getOrCreateAuthor("Kramer", "@kramer", kramerThumb);
long SECOND = 1000l;
long MINUTE = SECOND * 60;
long HOUR = MINUTE * 60;
long DAY = HOUR * 24;
long t = System.currentTimeMillis() - 2 * DAY;
out.add(createItem(george, new Date(t), "Now you can create CN1Libs with embedded CSS Styles", "https://www.codenameone.com/img/blog/css-header.jpg"));
out.add(createItem(george, new Date(t), "We now have a reliable way to avoid clipping the Notch and Task bar on the iPhone X.", "https://www.codenameone.com/img/blog/new-features.jpg"));
return out;
}
private SearchTabsViewModel createViewModel() {
SearchTabsViewModel out = new SearchTabsViewModel();
out.set(SearchTabsViewModel.forYou, createSection());
out.set(SearchTabsViewModel.fun, createSection());
out.set(SearchTabsViewModel.news, createSection());
out.set(SearchTabsViewModel.sports, createSection());
out.set(SearchTabsViewModel.trending, createTrendingSection());
return out;
}
private Entity getOrCreateAuthor(String name, String id, String iconUrl) {
if (authors.containsKey(id)) {
return authors.get(id);
}
Entity author = new TWTAuthor();
author.set(Thing.name, name);
author.set(Thing.identifier, id);
author.set(Thing.thumbnailUrl, iconUrl);
authors.put(id, author);
return author;
}
}
BASELINE, BOTTOM, BRB_CENTER_OFFSET, BRB_CONSTANT_ASCENT, BRB_CONSTANT_DESCENT, BRB_OTHER, CENTER, CROSSHAIR_CURSOR, DEFAULT_CURSOR, DRAG_REGION_IMMEDIATELY_DRAG_X, DRAG_REGION_IMMEDIATELY_DRAG_XY, DRAG_REGION_IMMEDIATELY_DRAG_Y, DRAG_REGION_LIKELY_DRAG_X, DRAG_REGION_LIKELY_DRAG_XY, DRAG_REGION_LIKELY_DRAG_Y, DRAG_REGION_NOT_DRAGGABLE, DRAG_REGION_POSSIBLE_DRAG_X, DRAG_REGION_POSSIBLE_DRAG_XY, DRAG_REGION_POSSIBLE_DRAG_Y, E_RESIZE_CURSOR, HAND_CURSOR, LEFT, MOVE_CURSOR, N_RESIZE_CURSOR, NE_RESIZE_CURSOR, NW_RESIZE_CURSOR, RIGHT, S_RESIZE_CURSOR, SE_RESIZE_CURSOR, SW_RESIZE_CURSOR, TEXT_CURSOR, TOP, W_RESIZE_CURSOR, WAIT_CURSOR
Constructor and Description |
---|
TabsEntityView(Entity entity,
ViewNode node) |
Modifier and Type | Method and Description |
---|---|
void |
commit() |
protected Tabs |
createTabs() |
Container |
getVerticalScroller()
For use with CollapsibleHeaderContainer.
|
Node |
getViewNode() |
void |
update() |
bind, bindImpl, deinitialize, findProperty, getEntity, initComponent, isBindOnPropertyChangeEvents, setBindOnPropertyChangeEvents, setEntity, unbind, unbindImpl
add, add, add, add, add, add, addAll, addComponent, addComponent, addComponent, addComponent, animateHierarchy, animateHierarchyAndWait, animateHierarchyFade, animateHierarchyFadeAndWait, animateLayout, animateLayoutAndWait, animateLayoutFade, animateLayoutFadeAndWait, animateUnlayout, animateUnlayoutAndWait, applyRTL, calcPreferredSize, cancelRepaints, clearClientProperties, constrainHeightWhenScrollable, constrainWidthWhenScrollable, contains, createAnimateHierarchy, createAnimateHierarchyFade, createAnimateLayout, createAnimateLayoutFade, createAnimateLayoutFadeAndWait, createAnimateMotion, createAnimateUnlayout, createReplaceTransition, dragInitiated, drop, encloseIn, encloseIn, findDropTargetAt, findFirstFocusable, fireClicked, flushReplace, forceRevalidate, getBottomGap, getChildrenAsList, getClosestComponentTo, getComponentAt, getComponentAt, getComponentCount, getComponentIndex, getGridPosX, getGridPosY, getLayout, getLayoutHeight, getLayoutWidth, getLeadComponent, getLeadParent, getResponderAt, getSafeAreaRoot, getScrollIncrement, getSideGap, getUIManager, initLaf, invalidate, isEnabled, isSafeArea, isSafeAreaRoot, isScrollableX, isScrollableY, isSelectableInteraction, iterator, iterator, keyPressed, keyReleased, layoutContainer, morph, morphAndWait, paint, paintComponentBackground, paintGlass, paramString, pointerPressed, refreshTheme, removeAll, removeComponent, replace, replace, replaceAndWait, replaceAndWait, replaceAndWait, revalidate, revalidateLater, revalidateWithAnimationSafety, scrollComponentToVisible, setCellRenderer, setEnabled, setLayout, setLeadComponent, setSafeArea, setSafeAreaRoot, setScrollable, setScrollableX, setScrollableY, setScrollIncrement, setShouldCalcPreferredSize, setShouldLayout, setUIManager, updateTabIndices
addDragFinishedListener, addDragOverListener, addDropListener, addFocusListener, addLongPressListener, addPointerDraggedListener, addPointerPressedListener, addPointerReleasedListener, addPullToRefresh, addScrollListener, addStateChangeListener, animate, bindProperty, blocksSideSwipe, calcScrollSize, contains, containsOrOwns, createStyleAnimation, deinitializeCustomStyle, dragEnter, dragExit, dragFinished, draggingOver, drawDraggedImage, focusGained, focusLost, getAbsoluteX, getAbsoluteY, getAllStyles, getAnimationManager, getBaseline, getBaselineResizeBehavior, getBindablePropertyNames, getBindablePropertyTypes, getBorder, getBoundPropertyValue, getBounds, getBounds, getClientProperty, getCloudBoundProperty, getCloudDestinationProperty, getComponentForm, getComponentState, getCursor, getDirtyRegion, getDisabledStyle, getDraggedx, getDraggedy, getDragImage, getDragRegionStatus, getDragSpeed, getEditingDelegate, getHeight, getInlineAllStyles, getInlineDisabledStyles, getInlinePressedStyles, getInlineSelectedStyles, getInlineStylesTheme, getInlineUnselectedStyles, getInnerHeight, getInnerPreferredH, getInnerPreferredW, getInnerWidth, getInnerX, getInnerY, getLabelForComponent, getName, getNativeOverlay, getNextFocusDown, getNextFocusLeft, getNextFocusRight, getNextFocusUp, getOuterHeight, getOuterPreferredH, getOuterPreferredW, getOuterWidth, getOuterX, getOuterY, getOwner, getParent, getPreferredH, getPreferredSize, getPreferredSizeStr, getPreferredTabIndex, getPreferredW, getPressedStyle, getPropertyNames, getPropertyTypeNames, getPropertyTypes, getPropertyValue, getSameHeight, getSameWidth, getScrollable, getScrollAnimationSpeed, getScrollDimension, getScrollOpacity, getScrollOpacityChangeSpeed, getScrollX, getScrollY, getSelectCommandText, getSelectedRect, getSelectedStyle, getStyle, getTabIndex, getTensileLength, getTextSelectionSupport, getTooltip, getUIID, getUnselectedStyle, getVisibleBounds, getVisibleBounds, getWidth, getX, getY, growShrink, handlesInput, hasFixedPreferredSize, hasFocus, hideNativeOverlay, initCustomStyle, installDefaultPainter, isAlwaysTensile, isBlockLead, isCellRenderer, isChildOf, isDragActivated, isDragAndDropOperation, isDraggable, isDragRegion, isDropTarget, isEditable, isEditing, isFlatten, isFocusable, isGrabsPointerEvents, isHidden, isHidden, isHideInLandscape, isHideInPortrait, isIgnorePointerEvents, isInClippingRegion, isInitialized, isOpaque, isOwnedBy, isRippleEffect, isRTL, isScrollable, isScrollVisible, isSetCursorSupported, isSmoothScrolling, isSnapToGrid, isStickyDrag, isTactileTouch, isTactileTouch, isTensileDragEnabled, isTraversable, isVisible, keyRepeated, laidOut, longKeyPress, longPointerPress, onScrollX, onScrollY, paintBackground, paintBackgrounds, paintBorder, paintBorderBackground, paintComponent, paintComponent, paintIntersectingComponentsAbove, paintLock, paintLockRelease, paintRippleOverlay, paintScrollbars, paintScrollbarX, paintScrollbarY, parsePreferredSize, pinch, pinchReleased, pointerDragged, pointerDragged, pointerHover, pointerHoverPressed, pointerHoverReleased, pointerPressed, pointerReleased, pointerReleased, putClientProperty, refreshTheme, refreshTheme, remove, removeDragFinishedListener, removeDragOverListener, removeDropListener, removeFocusListener, removeLongPressListener, removePointerDraggedListener, removePointerPressedListener, removePointerReleasedListener, removeScrollListener, removeStateChangeListener, repaint, repaint, requestFocus, resetFocusable, respondsToPointerEvents, scrollRectToVisible, scrollRectToVisible, setAlwaysTensile, setBlockLead, setBoundPropertyValue, setCloudBoundProperty, setCloudDestinationProperty, setComponentState, setCursor, setDirtyRegion, setDisabledStyle, setDraggable, setDropTarget, setEditingDelegate, setFlatten, setFocus, setFocusable, setGrabsPointerEvents, setHandlesInput, setHeight, setHidden, setHidden, setHideInLandscape, setHideInPortrait, setIgnorePointerEvents, setInitialized, setInlineAllStyles, setInlineDisabledStyles, setInlinePressedStyles, setInlineSelectedStyles, setInlineStylesTheme, setInlineUnselectedStyles, setIsScrollVisible, setLabelForComponent, setName, setNextFocusDown, setNextFocusLeft, setNextFocusRight, setNextFocusUp, setOpaque, setOwner, setPreferredH, setPreferredSize, setPreferredSizeStr, setPreferredTabIndex, setPreferredW, setPressedStyle, setPropertyValue, setRippleEffect, setRTL, setSameHeight, setSameSize, setSameWidth, setScrollAnimationSpeed, setScrollOpacityChangeSpeed, setScrollSize, setScrollVisible, setScrollX, setScrollY, setSelectCommandText, setSelectedStyle, setSize, setSmoothScrolling, setSnapToGrid, setTabIndex, setTactileTouch, setTensileDragEnabled, setTensileLength, setTooltip, setTraversable, setUIID, setUIID, setUnselectedStyle, setVisible, setWidth, setX, setY, shouldBlockSideSwipe, shouldRenderComponentSelection, showNativeOverlay, startEditingAsync, stopEditing, stripMarginAndPadding, styleChanged, toImage, toString, unbindProperty, updateNativeOverlay, visibleBoundsContains
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
forEach, spliterator
protected Tabs createTabs()
public void update()
update
in interface EntityView
public void commit()
commit
in interface EntityView
public Node getViewNode()
getViewNode
in interface EntityView
public Container getVerticalScroller()
For use with CollapsibleHeaderContainer. When tabs are used as the body it needs to let the collapsible header container which container us the current scrollable.
getVerticalScroller
in interface CollapsibleHeaderContainer.ScrollableContainer
Copyright © 2021. All Rights Reserved.